Hello everyone, in this article we will see the relationship between SharePoint 2013 and JavaScript from scratch to end.
You might be an expert in SharePoint or expert in JavaScript but still some people have doubt that, what’s this JavaScript doing inside the SharePoint. If you have that doubt too, then you are at the right place to kick that doubt out of your head. I know that, it is too late to share about basic stuff related to SP 2013 in the year 2016, but still it’s worth sharing.
Ok let’s get into the business. Microsoft has promoted JavaScript to a first-class language with the release of SharePoint 2013, so that all SharePoint Developers must be comfortable.
In SP 2013, not only the App-Model changed the development landscape but additional capabilities across the product make the JavaScript an even more important skill for SharePoint developers.
Please make a note of these good practices and learn the below stuffs before you are writing JavaScript programming in SharePoint, definitely it will be helpful:
JavaScript namespace – In JavaScript, by default all the code loaded into the memory are tossed into a single big bucket called Global Namespace. Every variables, every functions and everything go inside the Global Namespace. There might be a chance we run into the risk of having conflicts between all of these names. If you are using jQuery, make use of jquery.noconflict() mode.
JSON – An Important element to understand is JSON (JavaScript Object Notation). JSON is a subset of the whole JavaScript object literal notation. In real-world, it is more on Data-Exchange. These are Key-Value pairs, separated by commas and wrapped in curly braces.
Promises in JavaScript – Promises are pretty advanced topic in JavaScript, but it facilitate clean code when you’re doing asynchronous programming like almost everything we do in SharePoint JavaScript. It allow to control over asynchronous call and related interactions. Some of the important functions in Promises are,
.then – Take action upon success/failure of a function.
.always – This always gets called, whether it was a success or failure.
.when – wait until all actions complete before performing another action (it is non-blocking operation and it supports both success and fail triggering).
To know more about Promise please refer here.
Strict Mode – If you’re using JavaScript and not using strict mode it’s harder to guarantee that things will end up where you want them to end up. Strict mode enforces a better flavor for JavaScript, this is option explicit mode from VB. It is easy to setting up Strict Mode, just add literal string “use strict” to your code. It can be entered at Library level or Function level. It prevents a lots of dumb mistake (for example: var abc = “1”; // strict mode
abc = “1” // not strict mode
JavaScript Development Tools –
· Visual Studio Add-ons
o Web Essential 2012
o JavaScript Parser for Visual Studio (simple plugin used to find your way around large JavaScript files)
o JSLint for Visual Studio (code analysis for JavaScript).
· JSON Viewer
o https://jsonviewer.codeplex.com/
I am not explaining much on the above mentioned stuffs, because all those will lead to separate topic and it will divert from our main topic. So, I’m leaving it to the developers to learn by their own.
Out of the box SharePoint JavaScript environment:
1. SharePoint JavaScript
2. Variables
a. Context (ctx)
b. PageContext
3. Utilities
a. List Views
b. URL Manipulation
c. General Utilities
1. SharePoint JavaScript:
At a high level, SharePoint is just an ASP.Net web application like any other web application, it relies on client side scripting via JavaScript to deliver richer, more engaging user experiences. SharePoint has an extensive library of JavaScript used to deliver the experience we get out of the box. In the LAYOUTS directory there are around 170 JavaScript files.
The default SharePoint master page loads 5 js files (Core.js, Menu.js, Callout.js, Sharing.js, and SuiteLinks.js)

These files are loaded by using ScriptLink tag and using attribute OnDemand=”true”, so that these files are downloaded only if there’s a need.
Other common JavaScript libraries used across SharePoint include Init.js (more utility functions), SharePoint.js (main library for JavaScript Object Model – JSOM).
Many of the JavaScript files comes in SharePoint are in 2 flavors,
(*.js) Production and (*.debug.js) Debug.
Production – These files have the names what we would expect example: init.js, core.js etc and they are minified to reduce their size.
Debug – These have the same base name core, init, menu, whatever, but then debug.js instead of just .js so core.debug.js, menu.debug.js etc and these files that we want to work with if we need to debug.
2. Variables:
These variables represents an object that provides a lot of really useful information about the current context in which our code is running.
a. Context or ctx:
It provides wealth of information about the current page and the current environment. It won’t be available on all the pages, in general it’s not available on form pages (new, edit, display) and not available on layouts, site settings, administration pages etc. and also not available on apps unless we’re inside of a list. It is mainly available in Lists View pages.
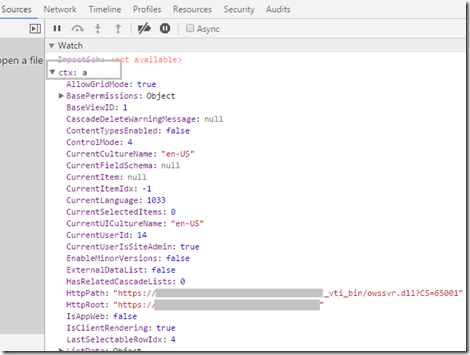
v This variable is read-only and updating this variable has no effect. Some of the useful properties of the context (ctx) object includes
Ø List Data – gives us information about the items shown in the ListView web part on the current page. The data will be available only when we are viewing a list.
i. List View only
ii. Row collection property:
1. Column values
2. Row is 0-based, based on display, not item ID
3. ContentTypeId
4. FSObjType(0=ListItem, 1=Folder)
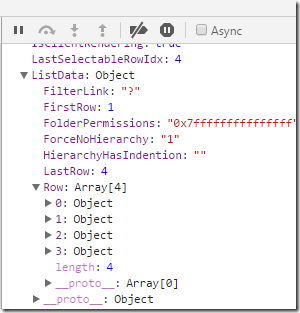
iii. Form URLs
1. displayFormURL
2. editFormURL
3. newFormURL
iv. ListTitle
v. ListURL
vi. ListGUID
vii. ListSchema
1. IsDocLib
2. Field Information
3. LocaleID
4. PagePath
viii. HttpRoot
1. BaseURL
ix. imagesPath
1. Relative Url to Images directory
It is good to make use of the Context (ctx) variable when there is only one ListView on the page.
b. _spPageContextInfo
It is pretty much similar to ctx variable and it is available on most of the pages like Layouts, site pages, apps etc. This provide access to lot of useful information, like current user, site, lists, pages etc.
Demo on SharePoint OOTB JavaScript Variables:
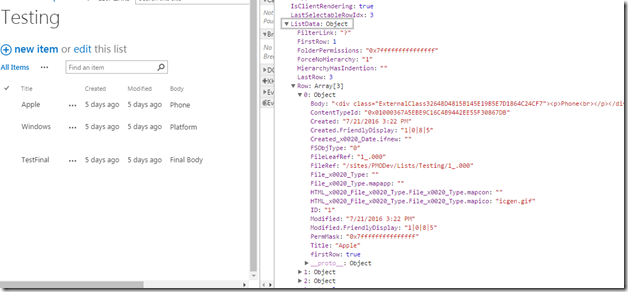
You can see lots of useful information available inside this ctx object, but I think that the most useful is if we need to go ahead and get the actual data values that are displayed inside the list view.
We do that inside the ListData Property, specifically inside the Row property of ListData. Each items are show inside this Row property in an array fashion.
document.location.href = SetUrlKeyValue("ID", "3", true, ctx.editFormUrl)
SetUrlKeyValue – This function allows us to specify the name or key value pair on the query string that we’re going to redirect the user.
In this example we want to specify the ID of 3, so that when we get the Edit form itself for that ID.
Here is the another example, with _spPageContextInfo variable
var verUI;
switch (_spPageContextInfo.webUIVersion) {
case 12:
verUI = "SharePoint 2007";
break;
case 14:
verUI = "SharePoint 2010";
break;
case 15:
verUI = "SharePoint 2013";
break;
default:
verUI = "Unknown";
break;
}
var msg = "Welcom to " + _spPageContextInfo.webTitle + ". You are browsing the site in " + _spPageContextInfo.currentCultureName + " using the " + verUI + " user interface";
alert(msg);
Output:
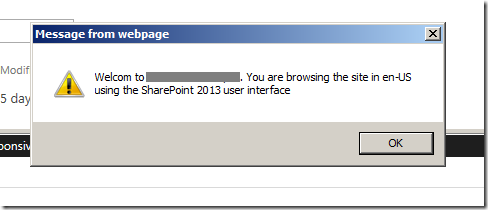
3. Utilities – List View OOTB functions:
SelectAllItems, DeselectAllItems, CountSelectedItems(ctx), SelectRowByIndex(ctx,idx,addToSelection), GetListItemByID(ctx, id)
Selecting Items which has Odd Item ID.
function selectItem() {
clearAll();
//Loop through each of the items using ctx.ListData.Row.length
for (var i = 0; i < ctx.ListData.Row.length; i++) {
//Selecting only odd ID number items
if (parseInt(ctx.ListData.Row[i].ID) % 2 !== 0) {
//Select that row using OOTB function
SelectRowByIndex(ctx, i, true);
}
}
}
function clearAll() {
var clvp = ctx.clvp;
var tab = clvp.tab;
//OOTB function to deselect the items
DeselectAllItems(ctx, tab.rows, false);
}
//Function to show the Item tab in the ribbon
function showItemTab() {
ribbon = SP.Ribbon.PageManager.get_instance().get_ribbon();
SelectRibbonTab("Ribbon.ListItem", true);
}
selectItem();
var itemCount = CountSelectedItems(ctx);
alert("There are " + itemCount + " selected items selected");
showItemTab();
var itemCount = CountSelectedItems(ctx);
alert("There are " + itemCount + " selected items selected");
showItemTab();
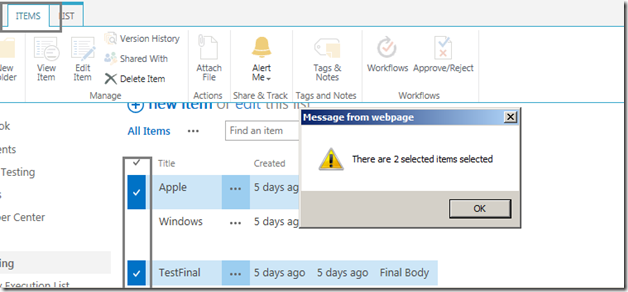
SP.Utilities.Utility object (sp.js) –
Few examples:
alert(SP.Utilities.Utility.getLayoutsPageUrl("SiteSetting.aspx"));
To get the layout page:
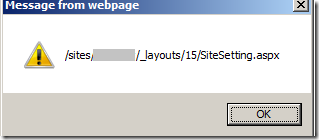
To navigate within the modal dialog box:
<a onclick=’SP.Utilities.HttpUtility.navigateTo(“url”)’> click to navigate inside </a>
To know more about this utility function please refer to this msdn link. We end this Part 1 here
Happy Coding
Ahamed
Leave a comment