In this article, we will see how to automate the build process once the code got checked in into TFS.
Automating build process and deployment process have many advantages. In this article let’s see how to automate build process; in the next article we will cover the continuous deployment process.
What is Continuous Integration?
Continuous Integration (CI) is the process of automating the build and testing of code every time a team member commits changes to version control. CI encourages developers to share their code and unit tests by merging their changes into a shared version control repository after every small task completion. Committing code triggers an automated build system to grab the latest code from the shared repository and to build, test, and validate the full master branch (also known as the trunk or main).
Continuous Integration Benefits
Ø Improve Developer Productivity
Ø Continuous integration helps your team be more productive by freeing developers from manual tasks and encouraging behaviors that help reduce the number of errors and bugs released to customers.
Ø Find and Address Bugs Earlier and Quicker.
Ø With more frequent testing, your team can discover and address bugs earlier before they grow into larger problems later.
Ø Deliver updates faster
Ø Continuous integration delivers updates to their customers faster and more frequently.
Prerequisites:
1. Make sure the user account that you’re going to use has permission to register the agent. Should be added in Agent Pool Administrators and Agent Pool Service Accounts.
2. Install Visual Studio/MS Build in TFS server to build the code in TFS server.
3. Install Microsoft Office Developer tools which will add SharePoint related DLLs in Build server.
4. Agent Pool Configuration
· Log on to the TFS server using the account for which you’ve prepared permissions as explained above.
· In your web browser, sign on to TFS, and navigate to the Agent pools tab:
URL:

5. Click Download agent.
6. On the Get agent dialog box, click Windows.
7. Click the Download button.
8. Extract the downloaded agent and run the Configure Agent in the command window by pointing to the extracted files location.
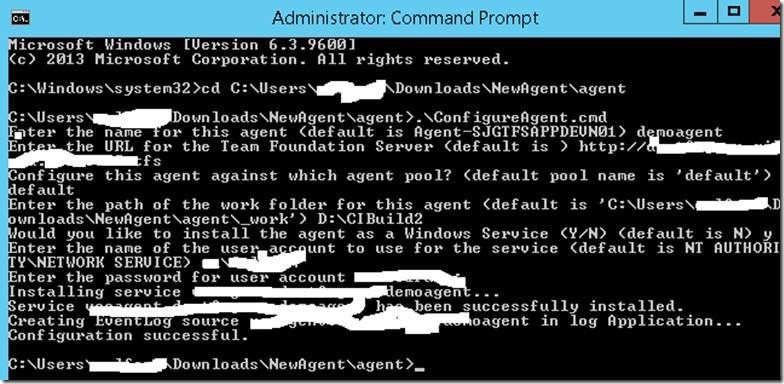
9. After agent pool creation, provide access to the required person by adding them in the Agent Pool Administrators and Agent Pool Service Accounts by selecting the Roles tab.
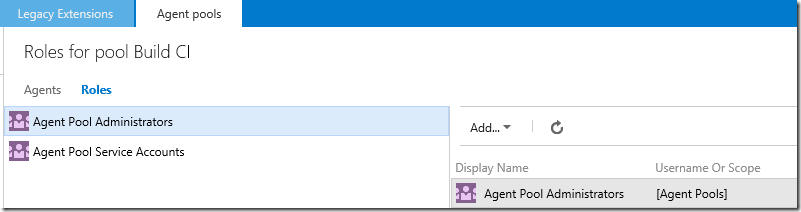
Create a Build Definition:
Below are the steps we need to follow to define the build.
- Open TFS url and navigate to Build tab and click on Create New Build Definition icon.
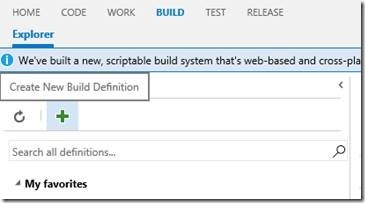
- Select Empty build definition in pop up.
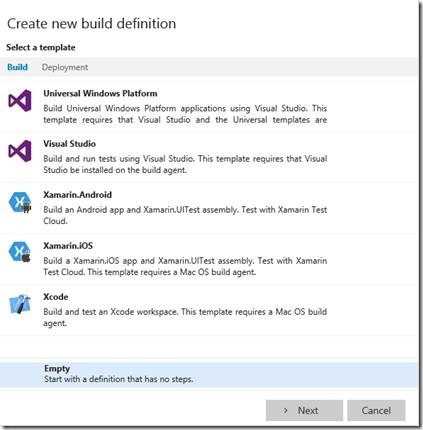
- Select the Continuous Integration Check Box and Choose the Repository and agent queue accordingly to create the build.
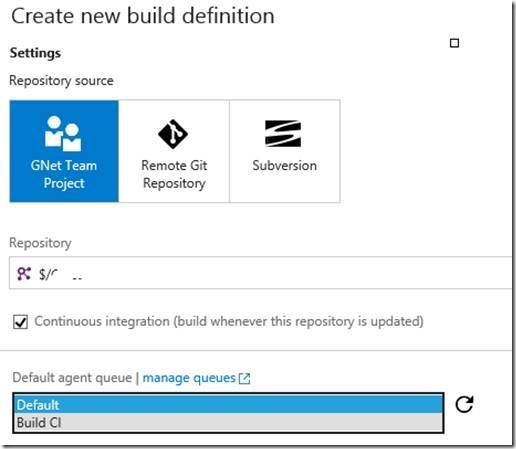
- In the Build Definition click on Add Build Step
- Add the Visual Studio Build step and provide configuration values as mentioned below.
Here, provide TFS solution path in Solution option, values provided for Platform and Configuration are Variabile to which we will assign value on Variables tab. Clean option is to clean then solution then it will build it.
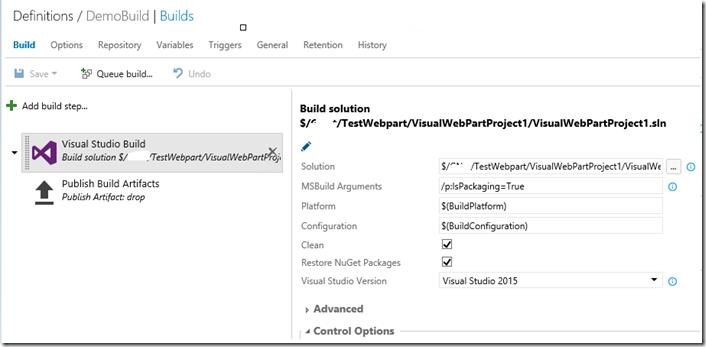
- Add Publish Build Artifacts and in that provide Drop folder location in which package will be captured. Publish Build Artifacts is to to publish the Artifacts in Drop folder which we will refere when deploying the solutin.
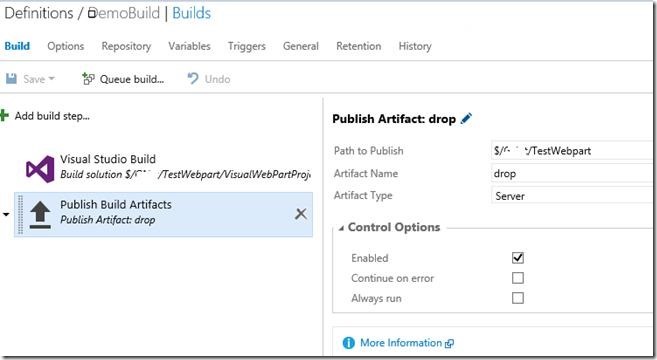
7. Under Repository tab, select true for Clean. This is to clean the repository before the build.
8. Set the variables as mentioned below.
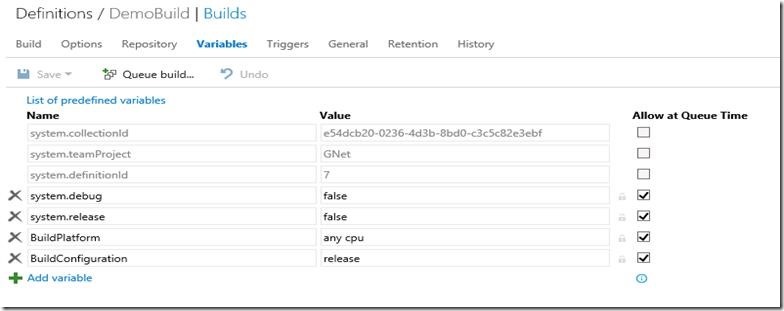
9. Save the Build Definition
Build the solution
1. Check in your solution into TFS.
2. Go to TFS and click on Build Tab.
3. In the Left hand side select the Build Definition using which you want to run the solution.
4. In the build definition, in Visual Studio step make sure you have selected the solution which you want to run.
5. Click on Queue build and then in the pop up click on OK.
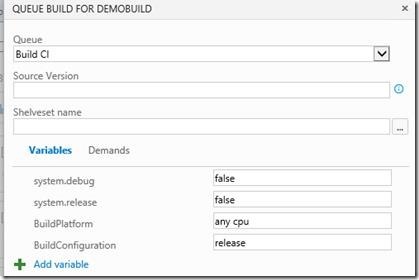
6. TFS will start building the solution; once it is done we will get the output screen as below.
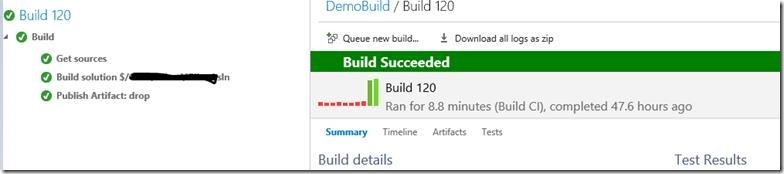
Common Build Issues
1. If we run SharePoint Server object Model code or code which refers OfficeDevPnp we will get below build error.
Solution: As we don’t have SharePoint related DLLs in Build server we will get this error. To solve this issue create a folder in C Drive and place all the necessary files there. Open the solution in Visual Studio Unload the solution then in csproj file in that provide hint path for the dll to which it is throwing error.

2. If there is no Visual Studio or .Net framework in the TFS servere, we will get below error message.
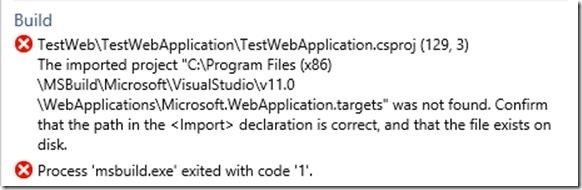
Installing Visual Studio/Appropriate MSBuild version on TFS server will resolve this issue.
Leave a comment